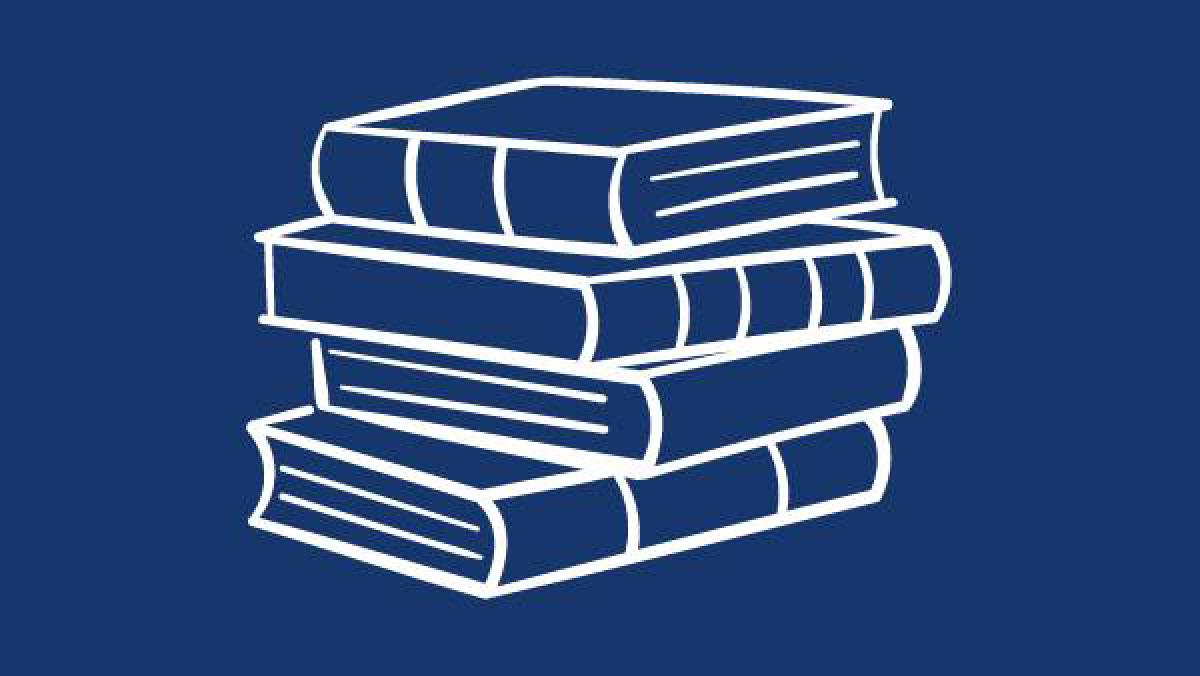
Tahap Preprocessing Data
Table of Contents
Penggunaan Python dalam Analisis Sentimen - This article is part of a series.
Pada tahap preprocessing data, kita melakukan serangkaian langkah untuk mempersiapkan teks agar siap digunakan dalam analisis sentimen. Proses ini membantu menghilangkan kekacauan dan memastikan data yang bersih untuk model analisis sentimen. Berikut adalah langkah-langkah preprocessing yang umumnya dilakukan:
A. Tokenisasi #
Tokenisasi adalah proses membagi teks menjadi unit-unit yang lebih kecil, seperti kata atau frasa.
from nltk.tokenize import word_tokenize
text = "This is an example sentence for tokenization."
tokens = word_tokenize(text)
print(tokens)
Hasil Print:
['This', 'is', 'an', 'example', 'sentence', 'for', 'tokenization', '.']
B. Pembersihan Teks #
Menghilangkan karakter khusus, tanda baca, dan elemen non-alfanumerik dari teks.
import re
text = "This is an example sentence with special characters! @#$"
cleaned_text = re.sub(r'[^A-Za-z0-9 ]+', '', text)
print(cleaned_text)
Hasil Print:
This is an example sentence with special characters
C. Case Folding #
Mengonversi seluruh teks ke huruf kecil untuk menghindari perbedaan dalam casing.
text = "This is an Example Sentence."
lowercased_text = text.lower()
print(lowercased_text)
Hasil Print:
this is an example sentence.
D. Hapus Stopwords #
Menghapus kata-kata umum (stopwords) yang tidak memberikan kontribusi signifikan pada analisis sentimen.
from nltk.corpus import stopwords
text = "This is a sample sentence with some common words."
stop_words = set(stopwords.words('english'))
filtered_tokens = [word for word in word_tokenize(text) if word.lower() not in stop_words]
print(filtered_tokens)
Hasil Print:
['This', 'sample', 'sentence', 'common', 'words', '.']
E. Stemming atau Lemmatization #
Mengonversi kata-kata ke bentuk dasar mereka (stems atau lemmas) untuk mengurangi variasi kata.
Implementasi dan Hasil Print (Stemming):
from nltk.stem import PorterStemmer
text = "Running, ran, runs, runner: they all involve running."
stemmer = PorterStemmer()
stemmed_words = [stemmer.stem(word) for word in word_tokenize(text)]
print(stemmed_words)
Hasil Print (Stemming):
['run', ',', 'ran', ',', 'run', ',', 'runner', ':', 'they', 'all', 'involv', 'run', '.']
Implementasi dan Hasil Print (Lemmatization):
from nltk.stem import WordNetLemmatizer
text = "Running, ran, runs, runner: they all involve running."
lemmatizer = WordNetLemmatizer()
lemmatized_words = [lemmatizer.lemmatize(word) for word in word_tokenize(text)]
print(lemmatized_words)
Hasil Print (Lemmatization):
['Running', ',', 'ran', ',', 'run', ',', 'runner', ':', 'they', 'all', 'involve', 'running', '.']
F. Pembentukan Fitur (Opsional) #
Mengubah teks menjadi representasi numerik, seperti TF-IDF atau vektor kata, agar dapat digunakan oleh model analisis sentimen.
from sklearn.feature_extraction.text import TfidfVectorizer
texts = ["This is the first document.", "This document is the second document.", "And this is the third one.", "Is this the first document?"]
vectorizer = TfidfVectorizer()
tfidf_matrix = vectorizer.fit_transform(texts)
print(tfidf_matrix)
Hasil Print:
(0, 5) 0.4387767428592343
(0, 4) 0.5419765697264572
(0, 3) 0.4387767428592343
(0, 2) 0.4387767428592343
(0, 1) 0.3587287382480895
(0, 0) 0.5419765697264572
(1, 5) 0.3587287382480895
(1, 4) 0.4415158946393502
(1, 3) 0.3587287382480895
(1, 2) 0.3587287382480895
(1, 0) 0.4415158946393502
(2, 5) 0.4387767428592343
(2, 4) 0.5419765697264572
(2, 3) 0.4387767428592343
(2, 2) 0.4387767428592343
(2, 1) 0.3587287382480895
(2, 0) 0.5419765697264572
(3, 5) 0.4387767428592343
(3, 4) 0.5419765697264572
(3, 3) 0.4387767428592343
(3, 2) 0.4387767428592343
(3, 1) 0.3587287382480895
(3, 0) 0.5419765697264572